2021.03.28 - [web/spring&spring boot] - [스프링부트] 게시판 - 게시글 등록 구현하기(1)
[스프링부트] 게시판 - 게시글 등록 구현하기(1)
사용자가 메인 페이지에서 글쓰기 버튼을 누르면 글 작성 페이지로 넘어가고 작성한 내용을 mysql 데이터베이스에 저장해봅시다. mysql 및 기본 프로젝트 설정은 이미 준비되어있다는 가정하에 진
coding-nyan.tistory.com
지난 시간에는 Entity만 구현하고 끝내버렸군요.
board domain
@Getter
@Entity
@NoArgsConstructor(access = AccessLevel.PROTECTED)
@EntityListeners(AuditingEntityListener.class)
public class Board{
@Id
@GeneratedValue
private Long id;
@Column(length = 10,nullable = false)
private String author;
@Column(length = 100,nullable = false)
private String title;
@Column(columnDefinition = "TEXT",nullable = false)
private String content;
@CreatedDate
@Column(updatable = false)
private LocalDateTime createdDate;
@LastModifiedDate
private LocalDateTime modifiedDate;
@Builder
public Board(Long id,String author,String title, String content) {
this.id = id;
this.author = author;
this.title = title;
this.content = content;
}
}
약간의 차이점이 생겼습니다. 글을 처음 생성한 시간과 수정 시간을 추가해봤어요. EntityListener를 추가한 경우에는 SpringApplication에 Auditing을 붙여줘야합니다.
@EnableJpaAuditing
@SpringBootApplication
public class BoardApplication {
public static void main(String[] args) {
SpringApplication.run(BoardApplication.class, args);
}
}
이렇게 말이죠. 혹은 JpaConfig 클래스를 만들어주고 거기에다가 @EnableJpaAuditing을 달아줘도 됩니다.
@Configuration
@EnableJpaAuditing
public class JpaConfig {
}
저는 어느 쪽이 더 적합한지 모르겠으니, 판단하시기에 적절한 것으로 선정해주세요.
Board Repository
public interface BoardRepository extends JpaRepository<Board,Long> {
}
JpaRepository를 상속받는 boardrepo 인터페이스를 만들어줍니다.
Board Service
@Service
public class BoardService {
private final BoardRepository boardRepository;
public BoardService(BoardRepository boardRepository){
this.boardRepository = boardRepository;
}
@Transactional
public Long savePost(BoardDto boardDto){
return boardRepository.save(boardDto.toEntity()).getId();
}
}
service는 repository 생성자 주입을 해줍니다. JPA의 경우 CRUD의 기본적인 내용들이 구현되어 있어서 불러다가 써주면 됩니다. 오늘은 게시글 등록, 즉 저장이므로 save를 호출해줄게요.
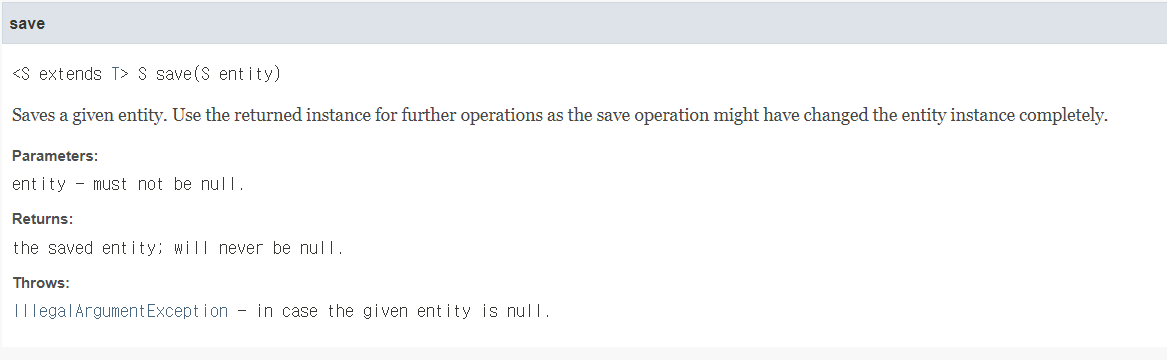
save의 경우 하나의 entity를 저장하는데 사용합니다. 그리고 저장된 entity를 반환해준다고 하네요. 만약에 여러 entity를 동시에 저장하고 싶은 경우에는 saveall을 사용해주세요. (iterable한 entity를 매개변수로 받음)
board Dto
@Getter
@Setter
@ToString
@NoArgsConstructor
public class BoardDto {
private Long id;
private String author;
private String title;
private String content;
private LocalDateTime createdDate;
private LocalDateTime modifiedDate;
public Board toEntity(){
Board build = Board.builder()
.id(id)
.author(author)
.title(title)
.content(content)
.build();
return build;
}
@Builder
public BoardDto(Long id,String author,String title,String content,LocalDateTime createdDate,LocalDateTime modifiedDate){
this.id = id;
this.author = author;
this.title = title;
this.content = content;
this.createdDate = createdDate;
this.modifiedDate = modifiedDate;
}
}
DTO는 Controller와 Service 사이에서 데이터를 주고받습니다.
BoardController
@PostMapping("/board/post")
public String save(BoardDto boardDto){
boardService.savePost(boardDto);
return "redirect:/board/list.html";
}
컨트롤러에 게시글 등록과 관련된 메서드를 만들어주세요. boardService도 생성자 주입을 해준뒤에 컨트롤러에서 boardService 인스턴스를 이용해서 JPA save를 간접 호출해줍니다.
이렇게 하면 얼추 게시글 등록 기능 구현은 마무리가 됩니다. 지금 작성한 코드는 컨트롤러 -> 서비스등이 연결되어 있는 상태인데 만약에 다른 서비스로 대체를 해야된다면 이게 또 컨트롤러의 코드도 같이 수정해줘야합니다. 이런 문제점을 어떻게 접근하면 해결할 수 있을까요?? 그에 대한 답은 나중에 다뤄보겠습니다.
소스 출처 :
https://kyuhyuk.kr/article/spring-boot/2020/07/19/Spring-Boot-JPA-MySQL-Board-Write-Post
[Spring Boot] 게시판 구현 하기 (1) - 글 작성 & 글 목록 출력
이번 시간에는 Spring Boot와 MySQL를 연동하고 게시판 기능의 글 작성과 글 목록 출력을 구현해보겠습니다.
kyuhyuk.kr
'web > spring&spring boot' 카테고리의 다른 글
[spring boot] 스프링 시큐리티 세션 활용하기 (0) | 2021.05.21 |
---|---|
[spring boot] 스프링 부트 게시판 글 조회 구현하기 (0) | 2021.05.20 |
[spring/spring boot] 스프링 부트 회원가입 후 자동로그인 기능 구현하기 (0) | 2021.05.17 |
[spring boot] 로그아웃 기능 구현하기 (0) | 2021.05.15 |
[spring boot] 로그인 기능 구현하기 (0) | 2021.04.25 |
2021.03.28 - [web/spring&spring boot] - [스프링부트] 게시판 - 게시글 등록 구현하기(1)
[스프링부트] 게시판 - 게시글 등록 구현하기(1)
사용자가 메인 페이지에서 글쓰기 버튼을 누르면 글 작성 페이지로 넘어가고 작성한 내용을 mysql 데이터베이스에 저장해봅시다. mysql 및 기본 프로젝트 설정은 이미 준비되어있다는 가정하에 진
coding-nyan.tistory.com
지난 시간에는 Entity만 구현하고 끝내버렸군요.
board domain
@Getter
@Entity
@NoArgsConstructor(access = AccessLevel.PROTECTED)
@EntityListeners(AuditingEntityListener.class)
public class Board{
@Id
@GeneratedValue
private Long id;
@Column(length = 10,nullable = false)
private String author;
@Column(length = 100,nullable = false)
private String title;
@Column(columnDefinition = "TEXT",nullable = false)
private String content;
@CreatedDate
@Column(updatable = false)
private LocalDateTime createdDate;
@LastModifiedDate
private LocalDateTime modifiedDate;
@Builder
public Board(Long id,String author,String title, String content) {
this.id = id;
this.author = author;
this.title = title;
this.content = content;
}
}
약간의 차이점이 생겼습니다. 글을 처음 생성한 시간과 수정 시간을 추가해봤어요. EntityListener를 추가한 경우에는 SpringApplication에 Auditing을 붙여줘야합니다.
@EnableJpaAuditing
@SpringBootApplication
public class BoardApplication {
public static void main(String[] args) {
SpringApplication.run(BoardApplication.class, args);
}
}
이렇게 말이죠. 혹은 JpaConfig 클래스를 만들어주고 거기에다가 @EnableJpaAuditing을 달아줘도 됩니다.
@Configuration
@EnableJpaAuditing
public class JpaConfig {
}
저는 어느 쪽이 더 적합한지 모르겠으니, 판단하시기에 적절한 것으로 선정해주세요.
Board Repository
public interface BoardRepository extends JpaRepository<Board,Long> {
}
JpaRepository를 상속받는 boardrepo 인터페이스를 만들어줍니다.
Board Service
@Service
public class BoardService {
private final BoardRepository boardRepository;
public BoardService(BoardRepository boardRepository){
this.boardRepository = boardRepository;
}
@Transactional
public Long savePost(BoardDto boardDto){
return boardRepository.save(boardDto.toEntity()).getId();
}
}
service는 repository 생성자 주입을 해줍니다. JPA의 경우 CRUD의 기본적인 내용들이 구현되어 있어서 불러다가 써주면 됩니다. 오늘은 게시글 등록, 즉 저장이므로 save를 호출해줄게요.
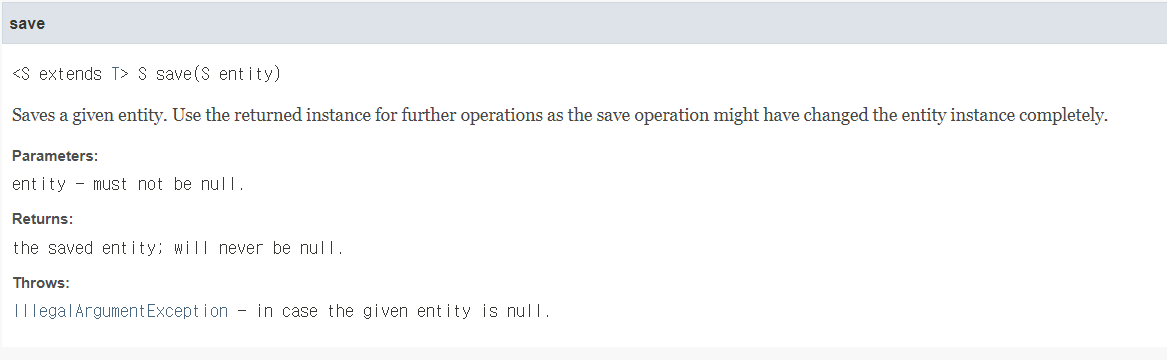
save의 경우 하나의 entity를 저장하는데 사용합니다. 그리고 저장된 entity를 반환해준다고 하네요. 만약에 여러 entity를 동시에 저장하고 싶은 경우에는 saveall을 사용해주세요. (iterable한 entity를 매개변수로 받음)
board Dto
@Getter
@Setter
@ToString
@NoArgsConstructor
public class BoardDto {
private Long id;
private String author;
private String title;
private String content;
private LocalDateTime createdDate;
private LocalDateTime modifiedDate;
public Board toEntity(){
Board build = Board.builder()
.id(id)
.author(author)
.title(title)
.content(content)
.build();
return build;
}
@Builder
public BoardDto(Long id,String author,String title,String content,LocalDateTime createdDate,LocalDateTime modifiedDate){
this.id = id;
this.author = author;
this.title = title;
this.content = content;
this.createdDate = createdDate;
this.modifiedDate = modifiedDate;
}
}
DTO는 Controller와 Service 사이에서 데이터를 주고받습니다.
BoardController
@PostMapping("/board/post")
public String save(BoardDto boardDto){
boardService.savePost(boardDto);
return "redirect:/board/list.html";
}
컨트롤러에 게시글 등록과 관련된 메서드를 만들어주세요. boardService도 생성자 주입을 해준뒤에 컨트롤러에서 boardService 인스턴스를 이용해서 JPA save를 간접 호출해줍니다.
이렇게 하면 얼추 게시글 등록 기능 구현은 마무리가 됩니다. 지금 작성한 코드는 컨트롤러 -> 서비스등이 연결되어 있는 상태인데 만약에 다른 서비스로 대체를 해야된다면 이게 또 컨트롤러의 코드도 같이 수정해줘야합니다. 이런 문제점을 어떻게 접근하면 해결할 수 있을까요?? 그에 대한 답은 나중에 다뤄보겠습니다.
소스 출처 :
https://kyuhyuk.kr/article/spring-boot/2020/07/19/Spring-Boot-JPA-MySQL-Board-Write-Post
[Spring Boot] 게시판 구현 하기 (1) - 글 작성 & 글 목록 출력
이번 시간에는 Spring Boot와 MySQL를 연동하고 게시판 기능의 글 작성과 글 목록 출력을 구현해보겠습니다.
kyuhyuk.kr
'web > spring&spring boot' 카테고리의 다른 글
[spring boot] 스프링 시큐리티 세션 활용하기 (0) | 2021.05.21 |
---|---|
[spring boot] 스프링 부트 게시판 글 조회 구현하기 (0) | 2021.05.20 |
[spring/spring boot] 스프링 부트 회원가입 후 자동로그인 기능 구현하기 (0) | 2021.05.17 |
[spring boot] 로그아웃 기능 구현하기 (0) | 2021.05.15 |
[spring boot] 로그인 기능 구현하기 (0) | 2021.04.25 |